php 常用方法 杂项啥都有
过滤掉 emoji 表情
// 过滤掉 emoji 表情
function filterEmoji($str)
{
if (is_array($str)) {
foreach ($str as $key => $value) {
$str[$key] = filterEmoji($value);
}
} else {
$str = preg_replace_callback(
'/./u',
function (array $match) {
return strlen($match[0]) >= 4 ? '' : $match[0];
}
,
$str
);
}
return $str;
}
用遮布把图片切成圆形
/**
* 用遮布把图片切成圆形
*
* @param $image mixed 图片路径/URL/二进制图片
* @param $diameter float 直径
* @return mixed
*/
function resize_to_circle($image, $diameter)
{
$diameter = ceil($diameter / 2) * 2;
if (!$image instanceof \Intervention\Image\Image) {
$client = new \GuzzleHttp\Client();
$image = $client->get($image);
$image = \Image::make($image->getBody()->getContents());
}
if ($image->width() > $image->height()) {
// 裁剪成正方形
$side_length = $image->height();
} else {
$side_length = $image->width();
}
$image->fit($side_length, $side_length);
$mask = \Image::canvas($side_length, $side_length);
// draw a white circle
$mask->circle($side_length - 2, $side_length / 2, $side_length / 2, function ($draw) {
$draw->background('#fff');
}
);
$image->mask($mask, false);
return $image->resize($diameter, null, function ($constraint) {
// 设定宽度是 $max_width,高度等比例双方缩放
$constraint->aspectRatio();
}
);
}
替换小程序二维码中间的 logo
/**
* 替换小程序二维码中间的 logo
*
* @param $img mixed 图片路径/URL/二进制图片
* @param $logo mixed 图片路径/URL/二进制图片
* @return mixed
*/
function replace_weapp_logo($img, $logo)
{
if (!$img instanceof \Intervention\Image\Image) {
$client = new \GuzzleHttp\Client();
$img = \Image::make($client->get($img)->getBody()->getContents());
}
$height = $img->height();
$circleImg = resize_to_circle($logo, $height / 2.22);
$circleHeight = $circleImg->height();
$y = intval(($height - $circleHeight) / 2);
$x = intval(($img->width() - $circleImg->width()) / 2);
$img->insert($circleImg, 'top-left', $x, $y);
return $img;
}
根据图片数组 拼接成九宫格式拼图
/**
* 根据图片数组 拼接成九宫格式拼图
*
* @param array $pic_list [带拼成的图片数组]
* @param integer $bg_w [背景图片宽度]
* @param string $format [阿里云图片获取参数]
* @return [type] [返回一个拼接好的图片(路径)]
*/
function mosaic_group_avatar($pic_list = array(), $bg_w = 396, $format = "")
{
$bg_h = $bg_w;
$pic_list = array_slice($pic_list, 0, 9);
// 只操作前9个图片
// 背景图
$background = \Image::canvas($bg_h, $bg_w, [255, 255, 255, 1]);
// 图片数量
$pic_count = count($pic_list);
$lineArr = array();
// 需要换行的位置
$space_x = 3;
$space_y = 3;
$line_x = 0;
switch ($pic_count) {
case 1: // 正中间
$start_x = intval($bg_w / 4);
// 开始位置X
$start_y = intval($bg_h / 4);
// 开始位置Y
$pic_w = intval($bg_w / 2);
// 宽度
$pic_h = intval($bg_h / 2);
// 高度
break;
case 2: // 中间位置并排
$start_x = 2;
$start_y = intval($bg_h / 4) + 3;
$pic_w = intval($bg_w / 2) - 5;
$pic_h = intval($bg_h / 2) - 5;
$space_x = 5;
break;
case 3:
$start_x = $bg_w / 4;
// 开始位置X
$start_y = 5;
// 开始位置Y
$pic_w = intval($bg_w / 2) - 5;
// 宽度
$pic_h = intval($bg_h / 2) - 5;
// 高度
$lineArr = array(2);
$line_x = 4;
break;
case 4:
$start_x = 4;
// 开始位置X
$start_y = 5;
// 开始位置Y
$pic_w = intval($bg_w / 2) - 5;
// 宽度
$pic_h = intval($bg_h / 2) - 5;
// 高度
$lineArr = array(3);
$line_x = 4;
break;
case 5:
$start_x = $bg_w / 6;
// 开始位置X
$start_y = $bg_h / 6;
// 开始位置Y
$pic_w = intval($bg_w / 3) - 5;
// 宽度
$pic_h = intval($bg_h / 3) - 5;
// 高度
$lineArr = array(3);
$line_x = 5;
break;
case 6:
$start_x = 5;
// 开始位置X
$start_y = $bg_h / 6;
// 开始位置Y
$pic_w = intval($bg_w / 3) - 5;
// 宽度
$pic_h = intval($bg_h / 3) - 5;
// 高度
$lineArr = array(4);
$line_x = 5;
break;
case 7:
$start_x = $bg_w / 3;
// 开始位置X
$start_y = 5;
// 开始位置Y
$pic_w = intval($bg_w / 3) - 5;
// 宽度
$pic_h = intval($bg_h / 3) - 5;
// 高度
$lineArr = array(2, 5);
$line_x = 5;
break;
case 8:
$start_x = $bg_w / 6;
// 开始位置X
$start_y = 5;
// 开始位置Y
$pic_w = intval($bg_w / 3) - 5;
// 宽度
$pic_h = intval($bg_h / 3) - 5;
// 高度
$lineArr = array(3, 6);
$line_x = 5;
break;
case 9:
$start_x = 5;
// 开始位置X
$start_y = 5;
// 开始位置Y
$pic_w = intval($bg_w / 3) - 5;
// 宽度
$pic_h = intval($bg_h / 3) - 5;
// 高度
$lineArr = array(4, 7);
$line_x = 5;
break;
}
$client = new \GuzzleHttp\Client();
foreach ($pic_list as $k => $pic_path) {
$kk = $k + 1;
if (in_array($kk, $lineArr)) {
$start_x = $line_x;
$start_y = $start_y + $pic_h + $space_y;
}
try {
$res = $client->get($pic_path . $format);
} catch (Exception $e) {
continue;
}
$avatar = \Image::make($res->getBody()->getContents());
$avatar->resize($pic_w, $pic_w);
// $start_x,$start_y copy图片在背景中的位置
// 0,0 被copy图片的位置 $pic_w,$pic_h copy后的高度和宽度
$background->insert($avatar, 'top-left', $start_x, $start_y);
$start_x = $start_x + $pic_w + $space_x;
}
// 保存图像为 $imagePath.'$fname'.'.jpg'
return $background;
}
今天是周几
function getTodayOfWeek($type = 1, $today = null)
{
if ($type == 1) {
$week = [
'星期日',
'星期一',
'星期二',
'星期三',
'星期四',
'星期五',
'星期六',
];
} else {
$week = ['周日', '周一', '周二', '周三', '周四', '周五', '周六'];
}
if (!$today) {
$today = today();
}
return $week[$today->dayOfWeek];
}
function getWeekName($day)
{
$week = [
'Sun' => '日',
'Mon' => '一',
'Tue' => '二',
'Wed' => '三',
'Thu' => '四',
'Fri' => '五',
'Sat' => '六',
];
if (!is_array($day)) {
return $week[$day] ?? '';
} else {
$weeks = [];
foreach ($day as $item) {
$weeks[] = $week[$item];
}
return implode(',', $weeks);
}
}
文件大小的字节转换
function get_format_size($size)
{
$size = $size / 1024;
if ($size <= 1024) {
$size = floor($size * 100) / 100;
return sprintf("%.2f", $size) . 'KB';
}
$size = $size / 1024;
if ($size <= 1024) {
$size = floor($size * 100) / 100;
return sprintf("%.2f", $size) . 'MB';
}
$size = $size / 1024;
$size = floor($size * 100) / 100;
return (sprintf("%.2f", $size)) . 'GB';
}
时间转换成日期 周几
function date_to_week($datetime)
{
$arr = ['周日', '周一', '周二', '周三', '周四', '周五', '周六'];
return $arr[date('w', strtotime($datetime))];
}
获取最近的时间关系
function time_ago($posttime)
{
//当前时间的时间戳
$nowtimes = strtotime(date('Y-m-d H:i:s'), time());
//之前时间参数的时间戳
$posttimes = strtotime($posttime);
//相差时间戳
$counttime = $nowtimes - $posttimes;
//进行时间转换
if ($counttime <= 10) {
return '刚刚';
} else if ($counttime > 10 && $counttime <= 30) {
return '刚才';
} else if ($counttime > 30 && $counttime <= 60) {
return '刚一会';
} else if ($counttime > 60 && $counttime <= 120) {
return '1分钟前';
} else if ($counttime > 120 && $counttime <= 180) {
return '2分钟前';
} else if ($counttime > 180 && $counttime < 3600) {
return intval(($counttime / 60)) . '分钟前';
} else if ($counttime >= 3600 && $counttime < 3600 * 24) {
return intval(($counttime / 3600)) . '小时前';
} else if ($counttime >= 3600 * 24 && $counttime < 3600 * 24 * 2) {
return '昨天';
} else if ($counttime >= 3600 * 24 * 2 && $counttime < 3600 * 24 * 3) {
return '前天';
} else if ($counttime >= 3600 * 24 * 3 && $counttime <= 3600 * 24 * 20) {
return intval(($counttime / (3600 * 24))) . '天前';
} else {
return $posttime;
}
}
金额转中文大写
/**
* 金额转中文大写
*
* @param mixed $amount
* @return string
*/
function rmb_capital($amount, $isFirstWord = false)
{
$capitalNumbers = [
'零', '壹', '贰', '叁', '肆', '伍', '陆', '柒', '捌', '玖',
];
$integerUnits = ['', '拾', '佰', '仟',];
$placeUnits = ['', '万', '亿', '兆',];
$decimalUnits = ['角', '分', '厘', '毫',];
$result = [];
$arr = explode('.', $amount);
$integer = trim($arr[0] ?? '', '-');
$decimal = $arr[1] ?? '';
if (!((int)$decimal)) {
$decimal = '';
}
// 转换整数部分
// 从个位开始
$integerNumbers = $integer ? array_reverse(str_split($integer)) : [];
$last = null;
foreach (array_chunk($integerNumbers, 4) as $chunkKey => $chunk) {
if (!((int)implode('', $chunk))) {
// 全是 0 则直接跳过
continue;
}
array_unshift($result, $placeUnits[$chunkKey]);
foreach ($chunk as $key => $number) {
// 去除重复 零,以及第一位的 零,类似:1002、110
if (!$number && (!$last || $key === 0)) {
$last = $number;
continue;
}
$last = $number;
// 类似 1022,中间的 0 是不需要 佰 的
if ($number) {
array_unshift($result, $integerUnits[$key]);
}
array_unshift($result, $capitalNumbers[$number]);
}
}
if (!$result) {
array_push($result, $capitalNumbers[0]);
}
array_push($result, '圆');
if (!$decimal) {
array_push($result, '整');
}
// 转换小数位
$decimalNumbers = $decimal ? str_split($decimal) : [];
foreach ($decimalNumbers as $key => $number) {
array_push($result, $capitalNumbers[$number]);
array_push($result, $decimalUnits[$key]);
}
if (strpos((string)$amount, '-') === 0) {
array_unshift($result, '负');
}
#
return $isFirstWord ? '人民币' . implode('', $result) : implode('', $result);
}
Buy me a cup of coffee 🙂
觉得对你有帮助,就给我打赏吧,谢谢!
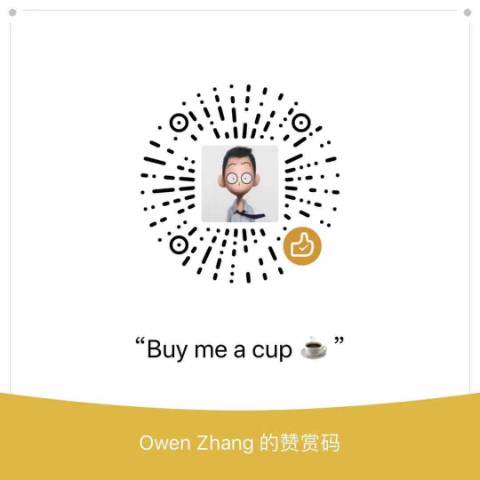